- Visual Basic Codes Pdf
- Free Visual Basic Code Examples
- Simple Visual Basic Code
- How To Code In Visual Basic
The program requires the user to enter the four values (Initial Balance, Annual Interest, Monthly Deposit, and Years of Investment). In turn, the program calculates the final balance. This calculation is known as the Future Value (FV) calculation, and Visual Basic.NET includes it as one of its built-in functions. The formula for Future Value is.
- Illustrates how to play a wave file in Visual Basic. It works by declaring a function to the WIN32 API sndPlaySound. Don't be intimidated: its two lines of code! Saul Greenberg: vbSketchpads: Illustrates two simple sketchpads.
- Public Class Form1 Private Sub btnCalculateClick (ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnCalculate.Click Dim principal, years As Integer Dim rate, interest, amount As Single principal = txtPrincipal.Text years = txtYears.Text rate = txtRate.Text If rbSimpleInterest.Checked = True Then interest = (principal. years. rate) / 100 Else amount = principal. Math.Pow ( (1 + rate / 100), years) interest = amount - principal End If txtInterest.Text =.
Home > Articles > Programming > Windows Programming
␡- Simple Calculations
Visual Basic Codes Pdf
This chapter is from the book
This chapter is from the book
Simple Calculations
Just having some values lying around does not make a program. You need to do something with the values. You can perform math on the values, or you can do something more substantial. Similarly, to make your programs more understandable, you will often need to use or create procedures. Visual Basic .NET will provide some of these procedures; you will write others. These procedures range from the operators, which provide many of the common mathematical calculations, to more complex functions that could affect strings or numbers.
Using Operators
In Visual Basic .NET, operators perform simple calculations and similar 'functions.' Most of the operators in Visual Basic .NET should be familiar to you as common algebraic symbols. However, some of them are unique to programming. Table 3.6 lists the most commonly used operators.
Table 3.6 Common Operators in Visual Basic .NET
Operator | Use | Example |
= | Assigns one value to another | X = 6 |
+ | Adds two values | Y = X + 7 (Y holds 13) |
- | Subtracts one value from another | Y = X – 4 (Y holds 2) |
* | Multiplies two values | Y = X * 2 (Y holds 12) |
/ | Divides one value by another | Y = X / 2 (Y holds 3) |
Divides one value by another, but only returns a whole number | Y = X 3 (Y holds 1) | |
Mod | Short for modulus; returns the remainder for a division | Y = X Mod 3 (Y holds 2) |
& | Combines two strings | S = 'Hello ' & 'World' (S holds 'Hello World') |
+= | Shorthand for adds a value and assigns the result | X += 2 (X holds 8) |
-= | Shorthand for subtracts a value and assigns the result | X –= 3 (X holds 5) |
*= | Shorthand for multiplies a value and assigns the result | X *= 6 (X holds 30) |
/= | Shorthand for divides by a value and assigns the result | X /= 5 (X holds 6) |
&= | Shorthand for combines with a string and assigns the result | S &= ', John' (S holds 'Hello World, John') |
^ | Raises one value to the power of an exponent | 3^4 (3 to the power of 4, returns 81) |
Built-In Functions
In addition to the functions provided by the .NET Framework, Visual Basic .NET has many built-in functions. These functions provide many useful capabilities, including conversion from one data type to another, mathematical calculations, string manipulation, and so on. You should know about some of these functions to be able to get around in Visual Basic .NET.
Conversion Functions
Some of the most important functions available to you in Visual Basic are the conversion functions. They enable you to take one type of data and convert it to another. With Visual Basic .NET, conversion functions have become even more important because this version of Visual Basic is much stricter about data types, and it does not automatically convert one type into another as previous versions did.
CAUTION
Free Visual Basic Code Examples
If you want Visual Basic .NET to automatically convert data types for you, you can turn off the strict type checking by adding Option Strict Off to the top of your files. You should know, however, that this could lead to unexpected results in your code (that is, bugs) if Visual Basic .NET converts a variable when you don't expect it to.
The conversion functions in Visual Basic .NET all begin with the letter 'C' (as in conversion), and end with an abbreviated form of the new type. In addition, there is a generic conversion function, CType, which can convert to any type. Table 3.7 describes the main conversion functions.
Table 3.7 Conversion Functions
Function | Description |
CBool | Converts to a Boolean. Anything that evaluates to False or 0 will be set to False; otherwise, it will be True. |
CByte | Converts to a Byte. Any value greater than 255, or any fractional information, will be lost. |
CChar | Converts to a single character. If the value is greater than 65,535, it will be lost. If you convert a String, only the first character is converted. |
CDate | Converts to a Date. One of the more powerful conversion functions, CDate can recognize some of the more common formats for entering a date. |
CDbl | Converts to a Double. |
CInt | Converts to an Integer. Fractions are rounded to the nearest value. |
CLng | Converts to a Long. Fractions are rounded to the nearest value. |
CSht | Converts to a Short. Fractions are rounded to the nearest value. |
CStr | Converts to a String. If the value is a Date, this will contain the Short Date format. |
CType | Converts to any type. This is a powerful function that enables you to convert any data type into any other type. Therefore, the syntax for this function is slightly different than the others. |
The syntax for CType is
in which oNewVariable and oOldVariable are placeholders for the variables that you're converting to and from, respectively. NewType is the type you are converting to. This can be any variable that you could put after the As in a declaration, so you can use this function to convert to enumerations, structures, and object types as well as simple types.
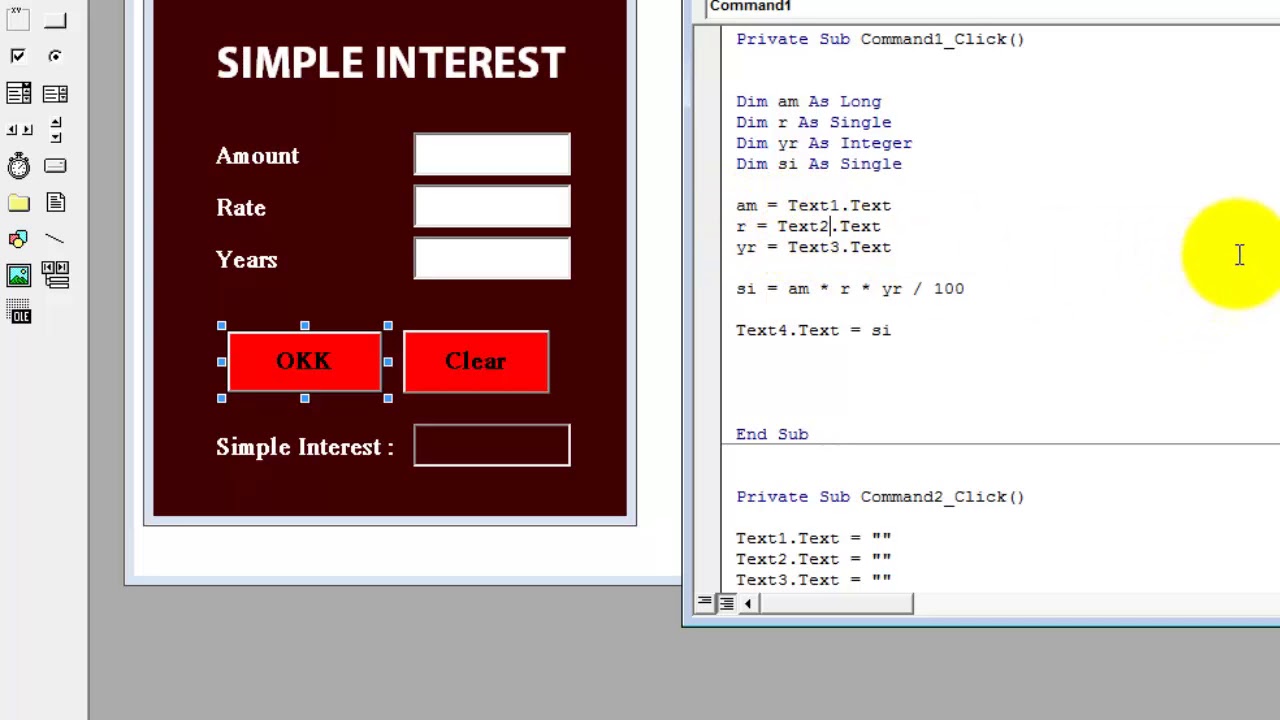
String Manipulation Functions
Most of Visual Basic's earlier string-related functions have been replaced in Visual Basic .NET with the functionality internal to the String class (we'll look at the String class in detail on Day 7). However, you might see some of the functions listed in Table 3.8 in older code, so you should be familiar with these functions.
Table 3.8 String-Handling Functions
Function | Description | Example |
Len | Returns the length of a string. | iValue = Len('Hello') ('iValue holds 5.) |
Chr | Returns the character based on the entered ASCII or Unicode value. | sValue = Chr(56) ('s Value holds the letter A.) |
Asc | Returns the ASCII or Unicode value. | iValue = Asc('A') ('iValue holds 56.) |
Left | Returns characters from a string, beginning with the leftmost character. Also requires the number of characters to return. | sValue = Left('Hello World', 2) ('sValue holds He.) |
Right | Returns characters from a string beginning with the rightmost character (the opposite of Left). Also requires the number of characters to return. | sValue =Right('Hello World',4) ('sValue holds orld.) |
Mid | Returns characters not at either end of a string. Mid returns any number of characters. The syntax for Mid is sReturn = Mid(String, Start, Length) in which Start is the character to begin returning from, and Length is the number of characters (including Start) to return. One nice feature is that if you omit Length, you will return all the characters from Starton. | sValue = Mid('Hello World', 4, 5)) ('sValue holds lo Wo.) sValue = Mid('Hello World', 7) ('sValue holds World.) |
Instr | Finds one string within another. This is useful when searching a file for some string. The syntax for the Instr function is iReturn = Instr(StartAtCharacter, SearchString, SearchFor, ComparisonType) | iValue =Instr(1,'Hello World','l')) ('iValue holds 3.) Keep in mind that the string you are searching for could be multiple characters, so a search for 'World', as in iValue = Instr(1, 'Hello World', 'World')) 'iValue holds 7.) |
StartAtCharacter is the position within the search string, SearchString, where the program begins searching (counting begins at 1). SearchString is the string to search in, and SearchFor is the string being sought. ComparisonType determines the case sensitivity of the search. If ComparisonTypeis set to 0 (Binary Compare), the search will be case sensitive. If it is ignored, or set to 1 (Text Compare), it will not be case sensitive. iReturn would then hold the position within the SearchString where the SearchFor begins. If the search for string is not found, iReturn will be 0. | ||
InstrRev searches from the right side of the string; otherwise, InstrRev is identical to Instr. InstrRev is useful when you are searching a string containing a directory path, and you want to view the lower directories first. iValue =InstrRev('Hello World', 'o')'iValue holds 8. | ||
LCase | Converts a string to all lowercase. | sValue = LCase('Hello World')'sValue holds hello world |
UCase | Converts a string to all uppercase. | sValue = UCase('Hello World')'sValue holds HELLO WORLD |
LTrim | Removes all leading spaces from a string | sValue = LTrim(' Hello World ') 'sValue holds 'Hello World ' |
RTrim | Removes all trailing spaces from a string | sValue = RTrim(' Hello World ') 'sValue holds ' Hello World' |
Trim | Removes all leading and trailing spaces from a string. | sValue = Trim(' Hello World ')sValue holds 'Hello World' |
Other Useful Functions
Finally, some generally useful functions don't fit into the other categories. These include functions that enable you to determine the type of a variable, as well as Date manipulation functions. Table 3.9 describes some of these functions.
Table 3.9 Miscellaneous Built-In Functions
Function | Description |
IsArray | Returns True if the parameter is an array. |
IsDate | Returns True if the parameter is recognizable as a date. |
IsNumeric | Returns True if the parameter is recognizable as a number. |
IsObject | Returns True if the parameter is some object type. |
TypeName | Returns the name of the data type of the parameter, for example, TypeName(sName) would return 'String'. |
Now | Returns the current date and time. |
Today | Returns the current date, with the time set to 0:00:00 a.m. (midnight). |
Simple Visual Basic Code
Related Resources
- Book $31.99
- eBook (Watermarked) $22.39
- Book $96.32
Visual Basic 6 is a third-generation event-driven programming language first released by Microsoft in 1991. In VB 6, there is no limit of what applications you could create, the sky is the limit. You can develop educational apps, financial apps, games, multimedia apps, animations, database applications and more.
The team at vbtutor.net has created many sample codes, please browse them on the sidebar. You are welcome to use the sample codes as a reference for your assignments and projects. However, the usage of the sample codes for commercial purposes without prior consent from the webmaster is strictly prohibited.
Visual Basic Sample Codes E-Book is written by our webmaster, Dr.Liew. It comprises 258 pages of captivating contents and 48 fascinating Sample Codes.Perfect source of reference for your VB projects. Check it out.
About Us
The Tutor and webmaster of Vbtutor.net, Dr.Liew Voon Kiong , holds a Bachelor's Degree in Mathematics, a Master's Degree in Management and a Doctoral Degree in Business Administration. He obtained the DBA degree from the University of South Australia.
He has been involved in programming for more than years. He created the popular online Visual Basic Tutorial in 1996 and since then the web site has attracted millions of visitors .It is the top-ranked Visual Basic tutorial website in many search engines including Google. Besides that, he has also written a few Visual Basic related books. One of the books, Visual Basic 6 Made Easy was published by Creativespace.com, an Amazon.com publisher.
The Slot Machine created using VB6
How To Code In Visual Basic
Copyright©2008 Dr.Liew Voon Kiong. All rights reserved |Contact|Privacy Policy